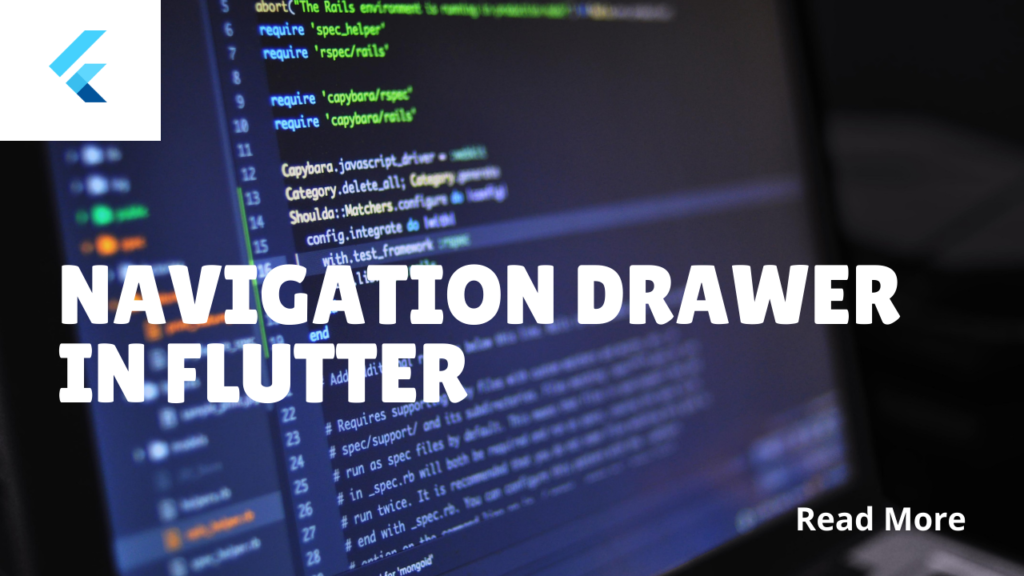
Hey coders, in this article we are going to talk about navigation drawer in flutter application. how we can implement navigation drawer same as Navigation drawer activity in Native Android. Actually compare to native android, building navigation drawer is quite easy in flutter project.
checkout compete step by step video tutorial
As shown in above video we are goin to create new widget for our Navigation drawer. create new file named nav_drawer.dart and inside navigation drawer file make state full widget and return Drawer() widget to show navigation drawer.
In side Drawer() widget we are going to create multiple menu items by using Listview() widget and for each item are going to use ListTile() widget.
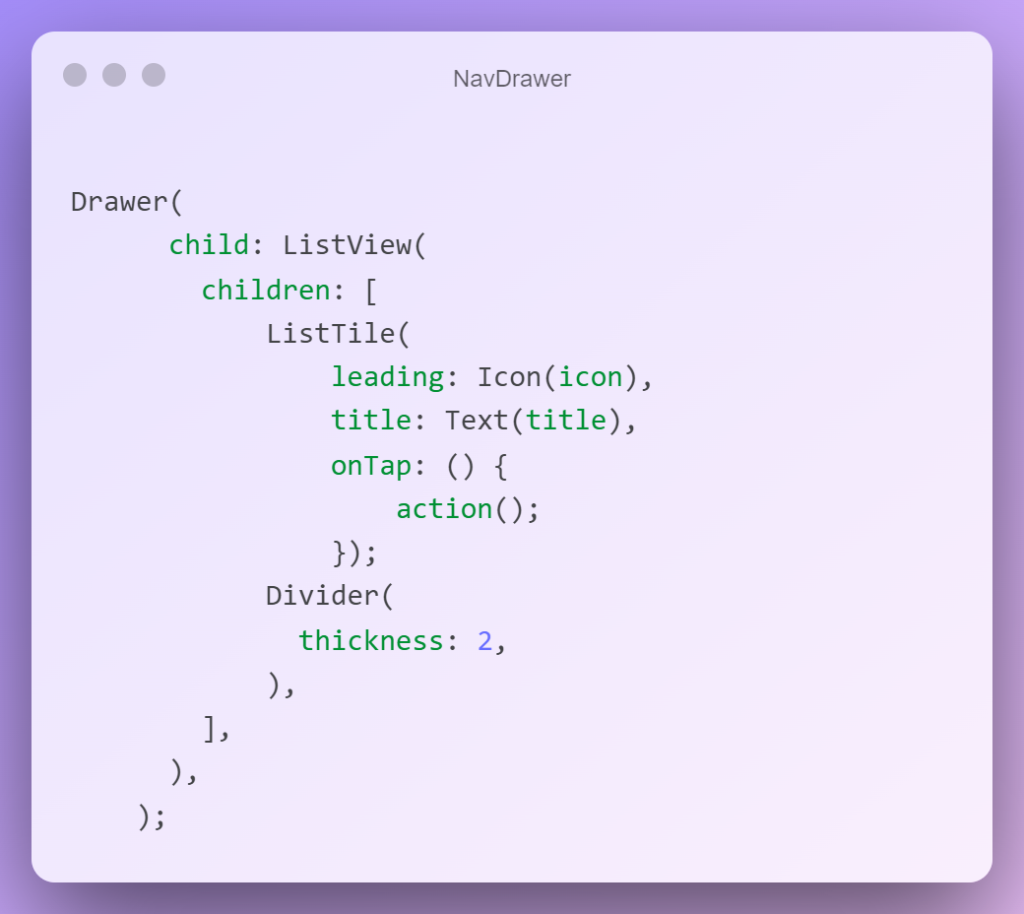
apply this NavDrawer widget inside your Homepage’s Scaffold() widget by passing named argument drawer
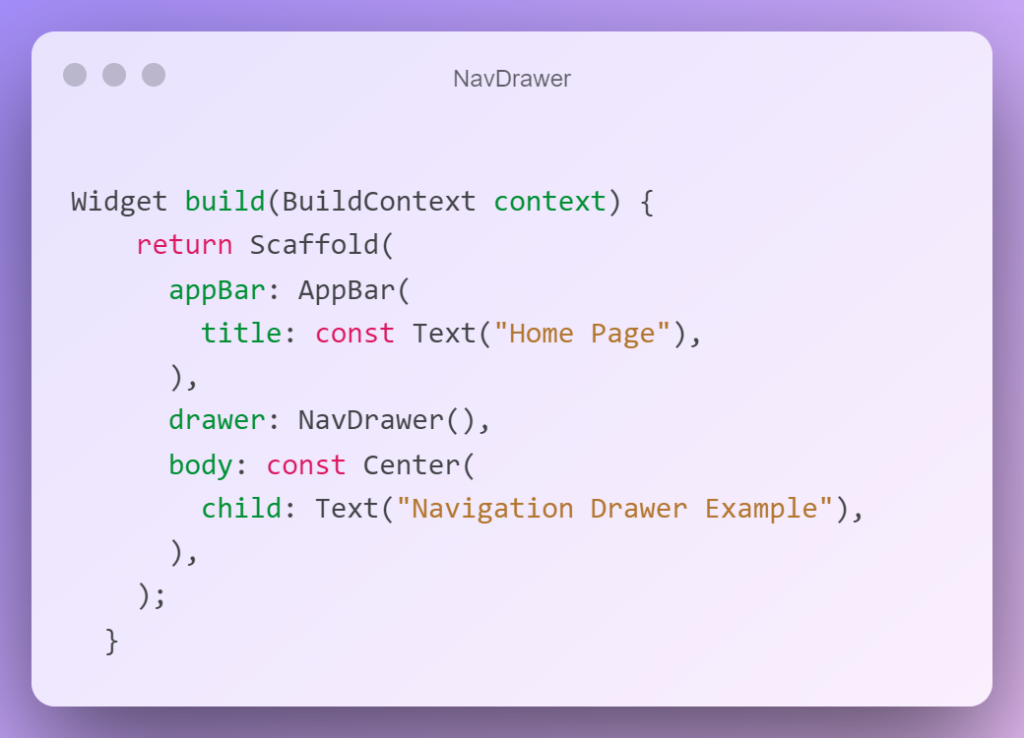
And that is it you can run your code and check your new navigation drawer working in your application.
here you can see the complete source code.
nav_drawer.dart
import 'package:flutter/material.dart';
import 'package:flutter_application_tutorial/contact.dart';
class NavDrawer extends StatefulWidget {
const NavDrawer({Key? key}) : super(key: key);
@override
_NavDrawerState createState() => _NavDrawerState();
}
class _NavDrawerState extends State<NavDrawer> {
@override
Widget build(BuildContext context) {
return Drawer(
child: ListView(
children: [
_getMenuItem(Icons.home, "Home", () {}),
Divider(
thickness: 2,
),
_getMenuItem(Icons.contact_page, "Contact", () {
Navigator.push(
context, MaterialPageRoute(builder: (context) => Contact()));
}),
Divider(
thickness: 2,
),
_getMenuItem(Icons.camera, "Camera", () {})
],
),
);
}
_getMenuItem(icon, title, Function action) {
return ListTile(
leading: Icon(icon),
title: Text(title),
onTap: () {
action();
});
}
}
Enjoy Coding 🙂